Add key-value pair to every object in array of objects in JavaScript
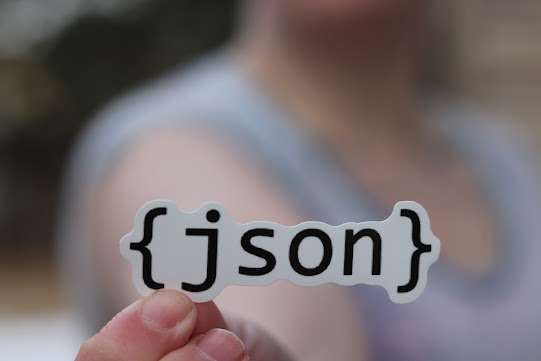
Sometimes, we've to add flag or some new key-value pair to every object in array of objects. For that we can follow three ways to achieve it. For suppose, we have an array of objects as below and we want to add gender key to all the objects students = [ { name: 'Radhika' , age: 26 , }, { name: 'Radha' , age: 24 , }, { name: 'Jyothi' , age: 25 , }, ]; // Expected Output students = [ { name: 'Radhika' , age: 26 , gender: 'female' }, { name: 'Radha' , age: 24 , gender: 'female' }, { name: 'Jyothi' , age: 25 , gender: 'female' }, ]; Method 1 - Using Map: //Here we have to assign to a variable to get the modified output. let response = this . students . map (( student ) => ({... student , gender: 'fe